Goal : 인터페이스란 무엇인지 알아보고, 코드에서는 어떻게 구현되는지 알아본다.
인터페이스란?
In Java, an interface is a blueprint or template of a class. It is much similar to the Java class but the only difference is that it has abstract methods and static constants.
There can be only abstract methods in an interface, that is there is no method body inside these abstract methods. The class that implements the interface should be abstract, otherwise, we need to define all the methods of the interface in the class.
자바에서 인터페이스는 클래스의 청사진이자 템플릿이다. 클래스와 비슷하지만 다른 점은 오로지 추상메소드와 정적 상수를 가진다는 것이다. 추상메소드만 존재하므로, 메소드의 바디는 없다. 인터페이스를 구현하는 클래스는 추상화를 해야하고 그게 아니라면 우리는 인터페이스의 메소드를 재정의를 해야한다.
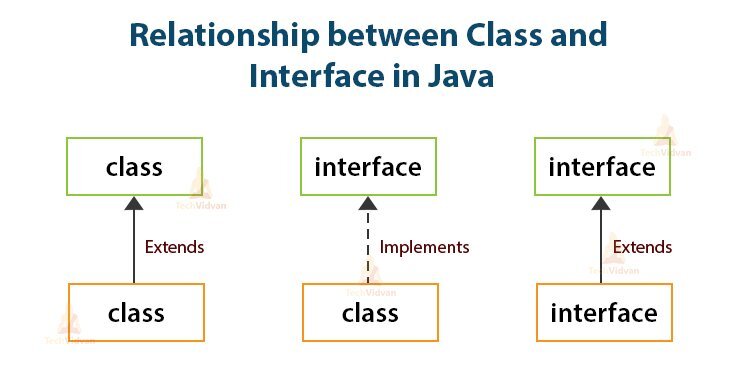
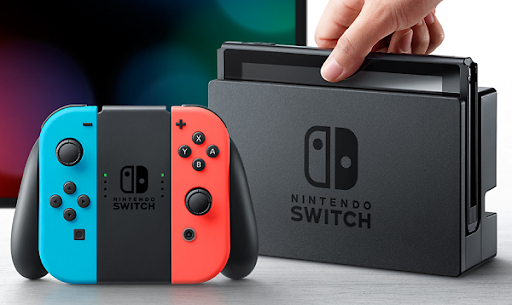
닌텐도 게임을 예로 들어보자. 일체형도 있지만 위의 사진에서는 칩(게임)을 꽂는 본체와 스위치 컨트롤러가 존재한다.
각자의 칩(슈퍼마리오 게임, 체스게임)을 넣으면 스위치 컨트롤러의 각 버튼은 각 게임에서 엄연히 다른 기능을 수행한다.
예를 들면, 마리오게임에서의 오른쪽 버튼은 10보 앞으로가 될 수 있지만, 체스게임에서의 오른쪽 버튼은 오른쪽 한칸 앞으로 가기이다.
이렇게 각 게임에서 다른 기능을 '스위치 컨트롤러'에서 미리 정의할 필요가 없고, '마리오게임'과 '체스게임'에서 그 기능을 구현하면 된다.
백문이 불여일타. 코드로 개념을 이해해보자!
예제 1
GamingConsole interface
public interface GamingConsole {
public void up();
public void down();
public void left();
public void right();
}
- 위 사진에서 스위치 컨트롤러가 될 것이며 위, 아래, 왼쪽, 오른쪽이라는 바디가 없는 메소드를 가지고 있다.
- interface라는 키워드를 사용한다.
ChessGame Class
public class ChessGame implements GamingConsole{
@Override
public void up() {
System.out.println("Move piece up");
}
@Override
public void down() {
System.out.println("Move piece down");
}
@Override
public void left() {
System.out.println("Move piece left");
}
@Override
public void right() {
System.out.println("Move piece right");
}
}
- 체스게임은 스위치 컨트롤러의 위, 아래, 왼쪽, 오른쪽메소드를 구현한다.
MarioGame Class
public class MarioGame implements GamingConsole{
@Override
public void up() {
System.out.println("jump");
}
@Override
public void down() {
System.out.println("goes into a hole");
}
@Override
public void left() {
}
@Override
public void right() {
System.out.println("go forward");
}
}
- 마리오게임은 스위치 컨트롤러의 위, 아래, 왼쪽, 오른쪽메소드를 구현한다.
GameRunner Class
public class GameRunner {
public static void main(String[] args){
//MarioGame marioGame = new MarioGame();
//ChessGame chessGame = new ChessGame();
GamingConsole marioGame = new MarioGame();
GamingConsole chessGame = new ChessGame();
marioGame.up();
marioGame.down();
marioGame.left();
marioGame.right();
System.out.println("--------------");
chessGame.up();
chessGame.down();
chessGame.left();
chessGame.right();
}
}
- MarioGame marioGame = new MarioGame(); 은 GamingConsole marioGame = new MarioGame(); 와 같다
- ChessGame chessGame = new ChessGame();은 GamingConsole chessGame = new ChessGame();와 같다
- 추상클래스는 인스턴스화를 할 수 없음과 같이 인터페이스도 그 자체를 인스턴스화를 할 수 없지만, 인터페이스를 구현한 클래스는 인터페이스 타입으로 변수를 선언하여 인스턴스를 생성할 수 있다.
- 인터페이스는 구현 코드가 없기 때문에 타입 상속이라고 한다.
결과
jump
goes into a hole
go forward
--------------
Move piece up
Move piece down
Move piece left
Move piece right
예제 2
ComplexAlgorithm interface
public interface ComplexAlgorithm {
int complexAlgorithm(int number1, int number2);
}
- 생성자 없음
DummyAlgorithm Class
public class DummyAlgorithm implements ComplexAlgorithm{
@Override
public int complexAlgorithm(int number1, int number2) {
return number1+number2;
}
}
- interface의 상속은 implements로 표현한다.
RealAlgorithm Class
public class RealAlgorithm implements ComplexAlgorithm{
@Override
public int complexAlgorithm(int number1, int number2) {
return number1*number2;
}
}
Project Class
public class Project {
public static void main(String[] args){
ComplexAlgorithm algorithm = new RealAlgorithm();
System.out.println(algorithm.complexAlgorithm(10,20));
}
}
결과
30
200
인터페이스 사용을 고려해야하는 경우
- 100% 추상화를 달성하고 싶을 때
- 다중 상속, 둘 이상의 인터페이스를 구현하려는 경우
- 누가 동작을 구현하는지에 관계없이 특정 데이터 유형의 동작을 지정하는 경우
추상클래스 vs 인터페이스
구분 | Abstract Class | Interfaces |
Keyword | abstract | interface |
변수 타입 | final, non-final, static and non-static 변수. | final and static 변수 |
final 변수 | final 변수로 선언 될 수 도 있고 아닐 수도 있음 | 변수는 기본적으로 final 선언됨 |
접근제한자 | public, protected, private and default. | public |
메소드 유형 | abstract and non-abstract or concrete methods. | 오로지 추상메소드만 java8 부터 non-static methods 지원 |
생성자 | o | x |
다중 상속 | x | o |
확장/구현 방법 | extends | implements |
속도 | 빠름 | 느림 |
사용시기 | 독립을 막기 위함 | 향후 개선 |
컴파일이 되었을 때 (.java->.class) 상수와 추상메서드로 자동 변환된다
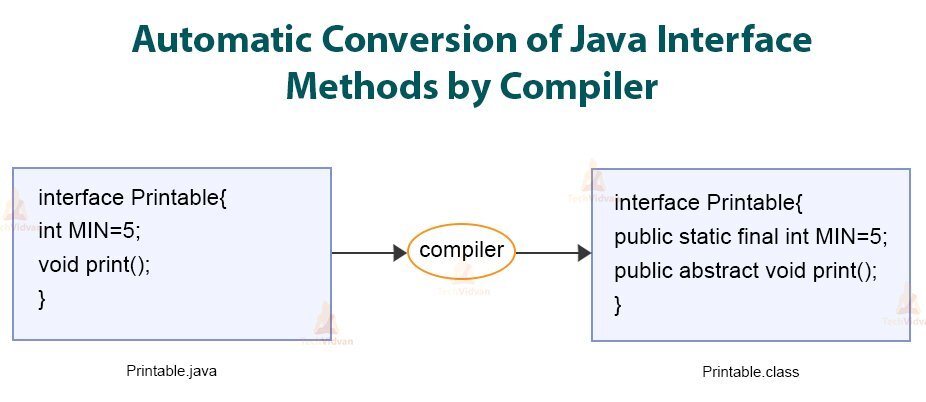
정리
인터페이스는 각 클래스에서 사용할 수 있는 공통적인 메소드를 제공한다. 그러나 그 메소드는 직접 구현해야함!
특징
- 멤버 변수는 항상 public static final이며 생략 가능하다.
- 멤버 메소드는 항상 public abstract 이며 생략 가능하다. (암시적으로 추상이므로 선언 시에 추상 키워드를 사용할 필요 x)
- 인터페이스 키워드를 사용해야 한다. interface
- 다중 구현이 가능하다. class A implements B,C,D
- 느슨한 결합
- 생성자 없음
- 인터페이스의 상속은 implements로 표현한다.
https://techvidvan.com/tutorials/java-abstract-class/ 그리고
@ The teacher Mr. Ranga 강의를 참조하고 있습니다.
'JAVA > 객체지향의 원리' 카테고리의 다른 글
[객체지향의 원리와 이해 2] 자바의 절차적 / 구조적 프로그래밍 (0) | 2023.06.26 |
---|---|
[객체지향의 원리와 이해 1] 사람을 사랑한 기술 (0) | 2023.06.26 |
[OOP] abstract / 추상화 (0) | 2022.09.05 |
[OOP] Inheritance / 상속 (0) | 2022.08.19 |
[OOP] Composition / 구성 (0) | 2022.08.15 |
Goal : 인터페이스란 무엇인지 알아보고, 코드에서는 어떻게 구현되는지 알아본다.
인터페이스란?
In Java, an interface is a blueprint or template of a class. It is much similar to the Java class but the only difference is that it has abstract methods and static constants.
There can be only abstract methods in an interface, that is there is no method body inside these abstract methods. The class that implements the interface should be abstract, otherwise, we need to define all the methods of the interface in the class.
자바에서 인터페이스는 클래스의 청사진이자 템플릿이다. 클래스와 비슷하지만 다른 점은 오로지 추상메소드와 정적 상수를 가진다는 것이다. 추상메소드만 존재하므로, 메소드의 바디는 없다. 인터페이스를 구현하는 클래스는 추상화를 해야하고 그게 아니라면 우리는 인터페이스의 메소드를 재정의를 해야한다.
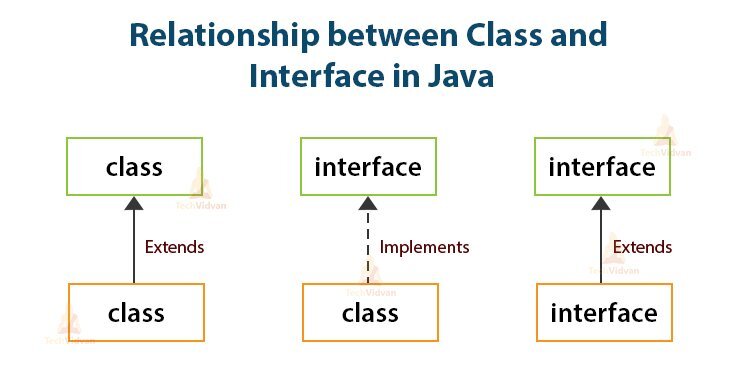
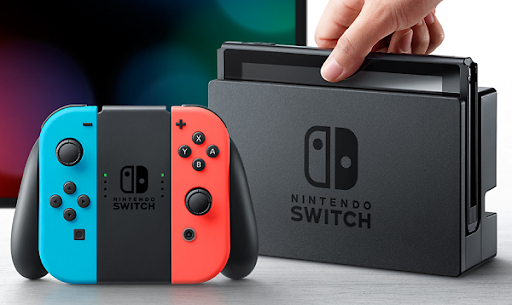
닌텐도 게임을 예로 들어보자. 일체형도 있지만 위의 사진에서는 칩(게임)을 꽂는 본체와 스위치 컨트롤러가 존재한다.
각자의 칩(슈퍼마리오 게임, 체스게임)을 넣으면 스위치 컨트롤러의 각 버튼은 각 게임에서 엄연히 다른 기능을 수행한다.
예를 들면, 마리오게임에서의 오른쪽 버튼은 10보 앞으로가 될 수 있지만, 체스게임에서의 오른쪽 버튼은 오른쪽 한칸 앞으로 가기이다.
이렇게 각 게임에서 다른 기능을 '스위치 컨트롤러'에서 미리 정의할 필요가 없고, '마리오게임'과 '체스게임'에서 그 기능을 구현하면 된다.
백문이 불여일타. 코드로 개념을 이해해보자!
예제 1
GamingConsole interface
public interface GamingConsole {
public void up();
public void down();
public void left();
public void right();
}
- 위 사진에서 스위치 컨트롤러가 될 것이며 위, 아래, 왼쪽, 오른쪽이라는 바디가 없는 메소드를 가지고 있다.
- interface라는 키워드를 사용한다.
ChessGame Class
public class ChessGame implements GamingConsole{
@Override
public void up() {
System.out.println("Move piece up");
}
@Override
public void down() {
System.out.println("Move piece down");
}
@Override
public void left() {
System.out.println("Move piece left");
}
@Override
public void right() {
System.out.println("Move piece right");
}
}
- 체스게임은 스위치 컨트롤러의 위, 아래, 왼쪽, 오른쪽메소드를 구현한다.
MarioGame Class
public class MarioGame implements GamingConsole{
@Override
public void up() {
System.out.println("jump");
}
@Override
public void down() {
System.out.println("goes into a hole");
}
@Override
public void left() {
}
@Override
public void right() {
System.out.println("go forward");
}
}
- 마리오게임은 스위치 컨트롤러의 위, 아래, 왼쪽, 오른쪽메소드를 구현한다.
GameRunner Class
public class GameRunner {
public static void main(String[] args){
//MarioGame marioGame = new MarioGame();
//ChessGame chessGame = new ChessGame();
GamingConsole marioGame = new MarioGame();
GamingConsole chessGame = new ChessGame();
marioGame.up();
marioGame.down();
marioGame.left();
marioGame.right();
System.out.println("--------------");
chessGame.up();
chessGame.down();
chessGame.left();
chessGame.right();
}
}
- MarioGame marioGame = new MarioGame(); 은 GamingConsole marioGame = new MarioGame(); 와 같다
- ChessGame chessGame = new ChessGame();은 GamingConsole chessGame = new ChessGame();와 같다
- 추상클래스는 인스턴스화를 할 수 없음과 같이 인터페이스도 그 자체를 인스턴스화를 할 수 없지만, 인터페이스를 구현한 클래스는 인터페이스 타입으로 변수를 선언하여 인스턴스를 생성할 수 있다.
- 인터페이스는 구현 코드가 없기 때문에 타입 상속이라고 한다.
결과
jump
goes into a hole
go forward
--------------
Move piece up
Move piece down
Move piece left
Move piece right
예제 2
ComplexAlgorithm interface
public interface ComplexAlgorithm {
int complexAlgorithm(int number1, int number2);
}
- 생성자 없음
DummyAlgorithm Class
public class DummyAlgorithm implements ComplexAlgorithm{
@Override
public int complexAlgorithm(int number1, int number2) {
return number1+number2;
}
}
- interface의 상속은 implements로 표현한다.
RealAlgorithm Class
public class RealAlgorithm implements ComplexAlgorithm{
@Override
public int complexAlgorithm(int number1, int number2) {
return number1*number2;
}
}
Project Class
public class Project {
public static void main(String[] args){
ComplexAlgorithm algorithm = new RealAlgorithm();
System.out.println(algorithm.complexAlgorithm(10,20));
}
}
결과
30
200
인터페이스 사용을 고려해야하는 경우
- 100% 추상화를 달성하고 싶을 때
- 다중 상속, 둘 이상의 인터페이스를 구현하려는 경우
- 누가 동작을 구현하는지에 관계없이 특정 데이터 유형의 동작을 지정하는 경우
추상클래스 vs 인터페이스
구분 | Abstract Class | Interfaces |
Keyword | abstract | interface |
변수 타입 | final, non-final, static and non-static 변수. | final and static 변수 |
final 변수 | final 변수로 선언 될 수 도 있고 아닐 수도 있음 | 변수는 기본적으로 final 선언됨 |
접근제한자 | public, protected, private and default. | public |
메소드 유형 | abstract and non-abstract or concrete methods. | 오로지 추상메소드만 java8 부터 non-static methods 지원 |
생성자 | o | x |
다중 상속 | x | o |
확장/구현 방법 | extends | implements |
속도 | 빠름 | 느림 |
사용시기 | 독립을 막기 위함 | 향후 개선 |
컴파일이 되었을 때 (.java->.class) 상수와 추상메서드로 자동 변환된다
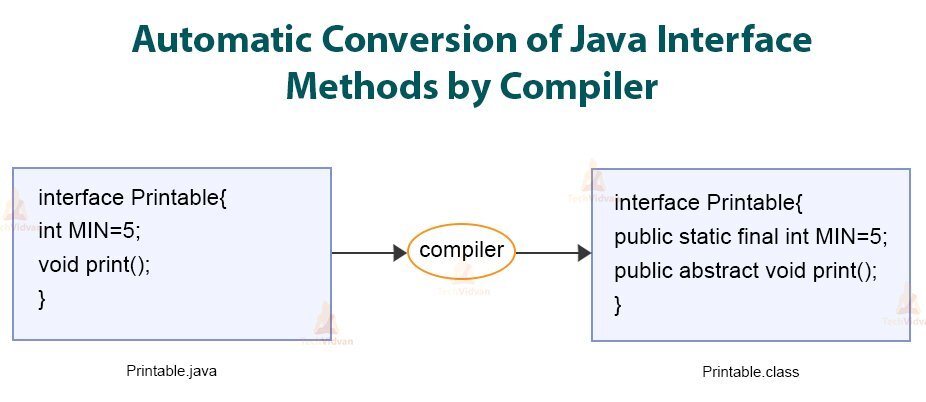
정리
인터페이스는 각 클래스에서 사용할 수 있는 공통적인 메소드를 제공한다. 그러나 그 메소드는 직접 구현해야함!
특징
- 멤버 변수는 항상 public static final이며 생략 가능하다.
- 멤버 메소드는 항상 public abstract 이며 생략 가능하다. (암시적으로 추상이므로 선언 시에 추상 키워드를 사용할 필요 x)
- 인터페이스 키워드를 사용해야 한다. interface
- 다중 구현이 가능하다. class A implements B,C,D
- 느슨한 결합
- 생성자 없음
- 인터페이스의 상속은 implements로 표현한다.
https://techvidvan.com/tutorials/java-abstract-class/ 그리고
@ The teacher Mr. Ranga 강의를 참조하고 있습니다.
'JAVA > 객체지향의 원리' 카테고리의 다른 글
[객체지향의 원리와 이해 2] 자바의 절차적 / 구조적 프로그래밍 (0) | 2023.06.26 |
---|---|
[객체지향의 원리와 이해 1] 사람을 사랑한 기술 (0) | 2023.06.26 |
[OOP] abstract / 추상화 (0) | 2022.09.05 |
[OOP] Inheritance / 상속 (0) | 2022.08.19 |
[OOP] Composition / 구성 (0) | 2022.08.15 |